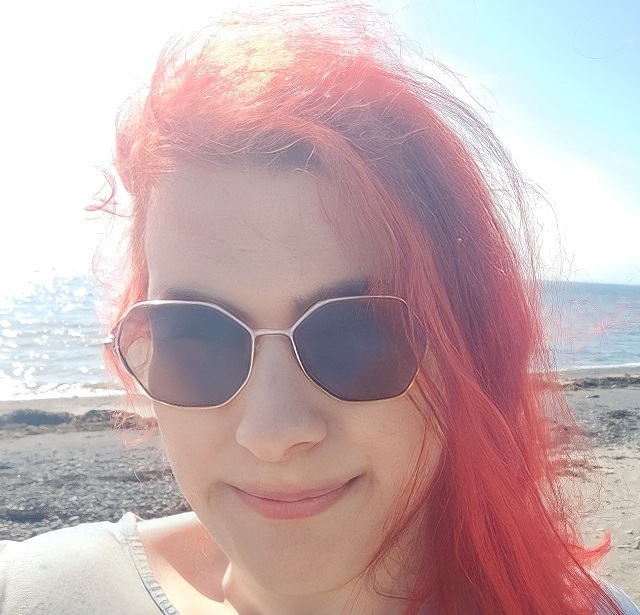
Neuron
A Haskell MNIST recognition neural network trainer and solver.
I implemented this a few years ago when I was trying to learn the basics of neural networks and machine learning. It's a feed-forward neural network, optimised using gradient descent. I also implemented an accelerated version using matrices and hmatrix.
It's quite pleasant to implement this kind of thing in haskell since a lot of the required calculations are implemented naturally as pure functions, and in some cases it's possible to use automatic differentation to derive the necessary derivatives.
Ocean simulation
This is an ocean I made in UnrealEngine 4.0 to try out material blueprints, which proved very powerful.
The entire ocean effect including the vertex displacement is done in a material blueprint and the buoyancy is simulated in C++.
Heterogeneous sparse voxel octree raytracing
This volume renderer was made as part of the dissertation I completed for my bachelors degree.
It first converts the scene into a sparse voxel octree format, which can then be traversed efficiently for real-time raytracing.
The transparency works by sampling iteratively at points through refractive mediums and the volume can even have a heterogeneous refractive index (i.e. it changes throughout the medium).
My dissertation itself can be read at the link above and the source code is also available.
Perspective/isometric interpolation
I created this effect for a game I was working on at the time.
Basically, the game can be viewed in both perspective and isometric views, and I desired a smooth transition when switching between them.
This makes use of the dolly pan technique and does the math to make it seamless.
Real-time mesh slicing
I needed a real-time mesh slicing effect for a project a friend was working on so I put together this test application to demonstrate the technique.
It works by first dividing the list of triangles in a mesh into two, one set for each side of the plane. For triangles that intersect the plane, it divides them and adds them to one side or the other.
This implementation is implemented on the CPU as a test but it should be heavily parallelisable.
Written in C++/OpenGL
3D Software renderer
I wrote this 3D software renderer when I was in university. I spent a lot of time optimizing it and adding features, and it was by far the fastest one in the class.
It supports hierarchical scenes and animations, and performs well for a software renderer, having been optimised to make use of SSE vector instructions using intrinsics. The code is a bit old and crazy now but I was proud of it at the time.
Written in C++